I can't understand why components with 'connect()' are stateful in react
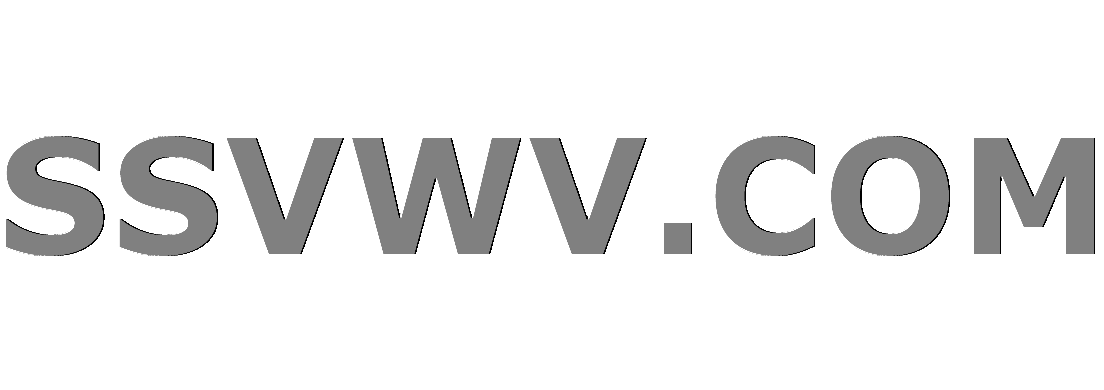
Multi tool use
My question is just same as the title.
Let's say I wrote the following code.
class TODOList extends Component {
render() {
const {todos, onClick} = this.props;
return (
<ul>
{todos.map(todo =>
<Todo
key={todo.id}
onClick={onClick}
{...todo}
/>
)}
</ul>
);
}
}
const mapStateToProps = (state) => {
return {
todos: state.todos
}
}
const mapDispatchToProps = (dispatch) => {
return {
onClick(data){
dispatch(complete(data))
}
}
}
export default connect(mapStateToProps,mapDispatchToProps)(TODOList);
Now, after the last line, this code will export the TODOList component with the state as props. It's not that it contains state, but just received state and will have them as 'props', just like the method name 'mapStateToProps' explains.
In the medium post(https://medium.com/@dan_abramov/smart-and-dumb-components-7ca2f9a7c7d0) written by Dan Abramov, container component handles data as state, and presentational property do as props. Isn't it a presentational component that deals with data as props? I'm stuck with the idea that the right container should be one like below.
class CommentList extends React.Component {
this.state = { comments: };
componentDidMount() {
fetchSomeComments(comments =>
this.setState({ comments: comments }));
}
render() {
return (
<ul>
{this.state.comments.map(c => (
<li>{c.body}—{c.author}</li>
))}
</ul>
);
}
}
I'm not sure why react-redux named the API 'mapStateToProps', when I tried to make 'stateful'(not handling data by property) container component
javascript reactjs redux react-redux
add a comment |
My question is just same as the title.
Let's say I wrote the following code.
class TODOList extends Component {
render() {
const {todos, onClick} = this.props;
return (
<ul>
{todos.map(todo =>
<Todo
key={todo.id}
onClick={onClick}
{...todo}
/>
)}
</ul>
);
}
}
const mapStateToProps = (state) => {
return {
todos: state.todos
}
}
const mapDispatchToProps = (dispatch) => {
return {
onClick(data){
dispatch(complete(data))
}
}
}
export default connect(mapStateToProps,mapDispatchToProps)(TODOList);
Now, after the last line, this code will export the TODOList component with the state as props. It's not that it contains state, but just received state and will have them as 'props', just like the method name 'mapStateToProps' explains.
In the medium post(https://medium.com/@dan_abramov/smart-and-dumb-components-7ca2f9a7c7d0) written by Dan Abramov, container component handles data as state, and presentational property do as props. Isn't it a presentational component that deals with data as props? I'm stuck with the idea that the right container should be one like below.
class CommentList extends React.Component {
this.state = { comments: };
componentDidMount() {
fetchSomeComments(comments =>
this.setState({ comments: comments }));
}
render() {
return (
<ul>
{this.state.comments.map(c => (
<li>{c.body}—{c.author}</li>
))}
</ul>
);
}
}
I'm not sure why react-redux named the API 'mapStateToProps', when I tried to make 'stateful'(not handling data by property) container component
javascript reactjs redux react-redux
"state" isn't a term specific to react, it's general to computer programming. It's a general term meaning remembered state of the system. en.wikipedia.org/wiki/State_(computer_science). Redux reducer state and component state are different things.
– Andy Ray
Dec 17 '18 at 2:26
add a comment |
My question is just same as the title.
Let's say I wrote the following code.
class TODOList extends Component {
render() {
const {todos, onClick} = this.props;
return (
<ul>
{todos.map(todo =>
<Todo
key={todo.id}
onClick={onClick}
{...todo}
/>
)}
</ul>
);
}
}
const mapStateToProps = (state) => {
return {
todos: state.todos
}
}
const mapDispatchToProps = (dispatch) => {
return {
onClick(data){
dispatch(complete(data))
}
}
}
export default connect(mapStateToProps,mapDispatchToProps)(TODOList);
Now, after the last line, this code will export the TODOList component with the state as props. It's not that it contains state, but just received state and will have them as 'props', just like the method name 'mapStateToProps' explains.
In the medium post(https://medium.com/@dan_abramov/smart-and-dumb-components-7ca2f9a7c7d0) written by Dan Abramov, container component handles data as state, and presentational property do as props. Isn't it a presentational component that deals with data as props? I'm stuck with the idea that the right container should be one like below.
class CommentList extends React.Component {
this.state = { comments: };
componentDidMount() {
fetchSomeComments(comments =>
this.setState({ comments: comments }));
}
render() {
return (
<ul>
{this.state.comments.map(c => (
<li>{c.body}—{c.author}</li>
))}
</ul>
);
}
}
I'm not sure why react-redux named the API 'mapStateToProps', when I tried to make 'stateful'(not handling data by property) container component
javascript reactjs redux react-redux
My question is just same as the title.
Let's say I wrote the following code.
class TODOList extends Component {
render() {
const {todos, onClick} = this.props;
return (
<ul>
{todos.map(todo =>
<Todo
key={todo.id}
onClick={onClick}
{...todo}
/>
)}
</ul>
);
}
}
const mapStateToProps = (state) => {
return {
todos: state.todos
}
}
const mapDispatchToProps = (dispatch) => {
return {
onClick(data){
dispatch(complete(data))
}
}
}
export default connect(mapStateToProps,mapDispatchToProps)(TODOList);
Now, after the last line, this code will export the TODOList component with the state as props. It's not that it contains state, but just received state and will have them as 'props', just like the method name 'mapStateToProps' explains.
In the medium post(https://medium.com/@dan_abramov/smart-and-dumb-components-7ca2f9a7c7d0) written by Dan Abramov, container component handles data as state, and presentational property do as props. Isn't it a presentational component that deals with data as props? I'm stuck with the idea that the right container should be one like below.
class CommentList extends React.Component {
this.state = { comments: };
componentDidMount() {
fetchSomeComments(comments =>
this.setState({ comments: comments }));
}
render() {
return (
<ul>
{this.state.comments.map(c => (
<li>{c.body}—{c.author}</li>
))}
</ul>
);
}
}
I'm not sure why react-redux named the API 'mapStateToProps', when I tried to make 'stateful'(not handling data by property) container component
javascript reactjs redux react-redux
javascript reactjs redux react-redux
edited Dec 17 '18 at 2:20


Mis94
1,14511026
1,14511026
asked Dec 16 '18 at 12:37


RheeRhee
1309
1309
"state" isn't a term specific to react, it's general to computer programming. It's a general term meaning remembered state of the system. en.wikipedia.org/wiki/State_(computer_science). Redux reducer state and component state are different things.
– Andy Ray
Dec 17 '18 at 2:26
add a comment |
"state" isn't a term specific to react, it's general to computer programming. It's a general term meaning remembered state of the system. en.wikipedia.org/wiki/State_(computer_science). Redux reducer state and component state are different things.
– Andy Ray
Dec 17 '18 at 2:26
"state" isn't a term specific to react, it's general to computer programming. It's a general term meaning remembered state of the system. en.wikipedia.org/wiki/State_(computer_science). Redux reducer state and component state are different things.
– Andy Ray
Dec 17 '18 at 2:26
"state" isn't a term specific to react, it's general to computer programming. It's a general term meaning remembered state of the system. en.wikipedia.org/wiki/State_(computer_science). Redux reducer state and component state are different things.
– Andy Ray
Dec 17 '18 at 2:26
add a comment |
3 Answers
3
active
oldest
votes
First of all these guidelines are not part of the bible
you should write code that is easy to reason about for YOU and your TEAM.
I think you are missing something, A redux Container is different than a react Container.
I mean, connect
will create the container for you, it doesn't mean the wraped component is a Container.
Basically you can export both versions from the same file, the Container (connected version) and the presentation version (the none connected one).
Another thing that usually throw people off, is the name of the function and argument of mapStateToProps
.
I prefer the name mapStoreToProps
as in
map the
redux
store to the component's props.
the name state
can be confusing when we are in the context of react
.
Edit
As a followup to your comment:
I totally didn't know these two are actually different. Could you please tell me about more details
They are different in the way that connect
is creating a "Container" for you.
connect
is a High Order Component that creates the Container Component for us with all the subscription logic + functions to pass portions of the store and action-creators to its children as props (mapStateToProps
& mapDispatchToProps
).
A "normal" Container is usually refers to a component that you write by hand, its often doesn't deal with how things should look but instead deal with certain logic of the app.
As for the other comments like
The connect HoC of react-redux just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified
As i mentioned above, this is partially true. It's not just injecting the properties into our component, its subscribing to the store, grabbing it from the Provider
(via context
) and its doing all these with optimizations in mind, so we won't have to do it by ourselves.
I'm not sure how mapStateToProps can confuse someone. We are talking about a state management library
I've seen some devs that misunderstood this because react
has a state
and redux
has a store
(at least that's how it was called in most of the tutorials and documentations).
this can be confusing to some people that are new to either react
or redux
.
Edit 2
It was a bit confusing due to the sentence 'it doesn't mean the wraped component is a Container.' Why is the wrapped component not a container? Isn't a component created by connect also a container?
I mean that the wrapped component that you wrote doesn't have to be a Container.
You can connect a "Presentation" component:
const Link = ({ active, children, onClick }) => {
if (active) {
return <span>{children}</span>
}
return (
<a
href=""
onClick={e => {
e.preventDefault()
onClick()
}}
>
{children}
</a>
)
}
// ...
export default connect(mapState, mapDispatch)(Link)
Thank you for your answer. But I don't understand this part : 'I think you are missing something, A redux Container is different than a react Container. I mean, connect will create the container for you, it doesn't mean the wraped component is a Container.' Yes, I totally didn't know these two are actually different. Could you please tell me about more details?
– Rhee
Dec 16 '18 at 13:04
I'm not sure howmapStateToProps
can confuse someone. We are talking about a state management library.
– Kyle Richardson
Dec 16 '18 at 13:05
1
Theconnect
HoC ofreact-redux
just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified.
– Kyle Richardson
Dec 16 '18 at 13:08
1
I've added some more details to answer some of the comments
– Sagiv b.g
Dec 16 '18 at 13:27
1
@Rhee I've answered you latest comment (Edit 2 section) Hope that's clarify it :)
– Sagiv b.g
Dec 16 '18 at 14:11
|
show 1 more comment
mapStateToProps
will be called when store data changes. It will pass the returned object as new props for the component. This will not affect the component's state. If you'd like to set a new state after the component got its new props you need to use another lifecycle method: static getDerivedStateFromProps
(in earlier versions of react componentWillRecieveProps
). The object returned by static getDerivedStateFromProps
will be your new state.
https://reactjs.org/docs/state-and-lifecycle.html#adding-lifecycle-methods-to-a-class
connect()
will connect your component to the redux store. Withouth the connect function (of course) your mapStateToProps
will not work.
I'm not sure why react-redux named the API 'mapStateToProps'
We are talking about the store's state :)
add a comment |
The high level purpose is to seamlessly integrate Redux's state management into the React application. Redux revolves around the store where all the state exists. There is no way to directly modify the store except through reducers whom receive actions from action creators and for that to happen we need for an action to be dispatched from the action creator.
The connect()
function directly connects our components to the Redux store by taking the state in the Redux store and mapping it into a prop
.
This is power of Redux and its why we use it.
Lets say you are building a component called LaundryList
and you want it to render a laundry list. After you have wired up the Provider
in your "parent" component, I put it in quotes because technically Provider
is a component so it becomes the parent.
You can then import the connect()
function from react-redux
, pass it mapStateToProps
in order to get that laundry list from the Redux store into your LaundryList
component.
Now that you have your list of linens inside of the LaundryList
component you can start to focus on building a list of elements out of them like so:
class LaundryList extends Component {
render() {
console.log(this.props.linens);
return <div>LaundryList</div>;
}
}
That contains the list of linens object and for every list of linens inside of there we are going to return some jsx that is going to represent that linen on my list.
Back inside my laundry list component I will add a helper method inside the laundry list component called render list like so:
class LaundryList extends Component {
renderList() {
}
render() {
return <div>LaundryList</div>;
}
}
So this purpose of this helper method is to take the list of linens, map over them and return a big blob of jsx like so:
class LaundryList extends Component {
renderList() {
return this.props.linens.map((linen) => {
return (
);
});
}
render() {
return <div>LaundryList</div>;
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53802181%2fi-cant-understand-why-components-with-connect-are-stateful-in-react%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
First of all these guidelines are not part of the bible
you should write code that is easy to reason about for YOU and your TEAM.
I think you are missing something, A redux Container is different than a react Container.
I mean, connect
will create the container for you, it doesn't mean the wraped component is a Container.
Basically you can export both versions from the same file, the Container (connected version) and the presentation version (the none connected one).
Another thing that usually throw people off, is the name of the function and argument of mapStateToProps
.
I prefer the name mapStoreToProps
as in
map the
redux
store to the component's props.
the name state
can be confusing when we are in the context of react
.
Edit
As a followup to your comment:
I totally didn't know these two are actually different. Could you please tell me about more details
They are different in the way that connect
is creating a "Container" for you.
connect
is a High Order Component that creates the Container Component for us with all the subscription logic + functions to pass portions of the store and action-creators to its children as props (mapStateToProps
& mapDispatchToProps
).
A "normal" Container is usually refers to a component that you write by hand, its often doesn't deal with how things should look but instead deal with certain logic of the app.
As for the other comments like
The connect HoC of react-redux just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified
As i mentioned above, this is partially true. It's not just injecting the properties into our component, its subscribing to the store, grabbing it from the Provider
(via context
) and its doing all these with optimizations in mind, so we won't have to do it by ourselves.
I'm not sure how mapStateToProps can confuse someone. We are talking about a state management library
I've seen some devs that misunderstood this because react
has a state
and redux
has a store
(at least that's how it was called in most of the tutorials and documentations).
this can be confusing to some people that are new to either react
or redux
.
Edit 2
It was a bit confusing due to the sentence 'it doesn't mean the wraped component is a Container.' Why is the wrapped component not a container? Isn't a component created by connect also a container?
I mean that the wrapped component that you wrote doesn't have to be a Container.
You can connect a "Presentation" component:
const Link = ({ active, children, onClick }) => {
if (active) {
return <span>{children}</span>
}
return (
<a
href=""
onClick={e => {
e.preventDefault()
onClick()
}}
>
{children}
</a>
)
}
// ...
export default connect(mapState, mapDispatch)(Link)
Thank you for your answer. But I don't understand this part : 'I think you are missing something, A redux Container is different than a react Container. I mean, connect will create the container for you, it doesn't mean the wraped component is a Container.' Yes, I totally didn't know these two are actually different. Could you please tell me about more details?
– Rhee
Dec 16 '18 at 13:04
I'm not sure howmapStateToProps
can confuse someone. We are talking about a state management library.
– Kyle Richardson
Dec 16 '18 at 13:05
1
Theconnect
HoC ofreact-redux
just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified.
– Kyle Richardson
Dec 16 '18 at 13:08
1
I've added some more details to answer some of the comments
– Sagiv b.g
Dec 16 '18 at 13:27
1
@Rhee I've answered you latest comment (Edit 2 section) Hope that's clarify it :)
– Sagiv b.g
Dec 16 '18 at 14:11
|
show 1 more comment
First of all these guidelines are not part of the bible
you should write code that is easy to reason about for YOU and your TEAM.
I think you are missing something, A redux Container is different than a react Container.
I mean, connect
will create the container for you, it doesn't mean the wraped component is a Container.
Basically you can export both versions from the same file, the Container (connected version) and the presentation version (the none connected one).
Another thing that usually throw people off, is the name of the function and argument of mapStateToProps
.
I prefer the name mapStoreToProps
as in
map the
redux
store to the component's props.
the name state
can be confusing when we are in the context of react
.
Edit
As a followup to your comment:
I totally didn't know these two are actually different. Could you please tell me about more details
They are different in the way that connect
is creating a "Container" for you.
connect
is a High Order Component that creates the Container Component for us with all the subscription logic + functions to pass portions of the store and action-creators to its children as props (mapStateToProps
& mapDispatchToProps
).
A "normal" Container is usually refers to a component that you write by hand, its often doesn't deal with how things should look but instead deal with certain logic of the app.
As for the other comments like
The connect HoC of react-redux just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified
As i mentioned above, this is partially true. It's not just injecting the properties into our component, its subscribing to the store, grabbing it from the Provider
(via context
) and its doing all these with optimizations in mind, so we won't have to do it by ourselves.
I'm not sure how mapStateToProps can confuse someone. We are talking about a state management library
I've seen some devs that misunderstood this because react
has a state
and redux
has a store
(at least that's how it was called in most of the tutorials and documentations).
this can be confusing to some people that are new to either react
or redux
.
Edit 2
It was a bit confusing due to the sentence 'it doesn't mean the wraped component is a Container.' Why is the wrapped component not a container? Isn't a component created by connect also a container?
I mean that the wrapped component that you wrote doesn't have to be a Container.
You can connect a "Presentation" component:
const Link = ({ active, children, onClick }) => {
if (active) {
return <span>{children}</span>
}
return (
<a
href=""
onClick={e => {
e.preventDefault()
onClick()
}}
>
{children}
</a>
)
}
// ...
export default connect(mapState, mapDispatch)(Link)
Thank you for your answer. But I don't understand this part : 'I think you are missing something, A redux Container is different than a react Container. I mean, connect will create the container for you, it doesn't mean the wraped component is a Container.' Yes, I totally didn't know these two are actually different. Could you please tell me about more details?
– Rhee
Dec 16 '18 at 13:04
I'm not sure howmapStateToProps
can confuse someone. We are talking about a state management library.
– Kyle Richardson
Dec 16 '18 at 13:05
1
Theconnect
HoC ofreact-redux
just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified.
– Kyle Richardson
Dec 16 '18 at 13:08
1
I've added some more details to answer some of the comments
– Sagiv b.g
Dec 16 '18 at 13:27
1
@Rhee I've answered you latest comment (Edit 2 section) Hope that's clarify it :)
– Sagiv b.g
Dec 16 '18 at 14:11
|
show 1 more comment
First of all these guidelines are not part of the bible
you should write code that is easy to reason about for YOU and your TEAM.
I think you are missing something, A redux Container is different than a react Container.
I mean, connect
will create the container for you, it doesn't mean the wraped component is a Container.
Basically you can export both versions from the same file, the Container (connected version) and the presentation version (the none connected one).
Another thing that usually throw people off, is the name of the function and argument of mapStateToProps
.
I prefer the name mapStoreToProps
as in
map the
redux
store to the component's props.
the name state
can be confusing when we are in the context of react
.
Edit
As a followup to your comment:
I totally didn't know these two are actually different. Could you please tell me about more details
They are different in the way that connect
is creating a "Container" for you.
connect
is a High Order Component that creates the Container Component for us with all the subscription logic + functions to pass portions of the store and action-creators to its children as props (mapStateToProps
& mapDispatchToProps
).
A "normal" Container is usually refers to a component that you write by hand, its often doesn't deal with how things should look but instead deal with certain logic of the app.
As for the other comments like
The connect HoC of react-redux just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified
As i mentioned above, this is partially true. It's not just injecting the properties into our component, its subscribing to the store, grabbing it from the Provider
(via context
) and its doing all these with optimizations in mind, so we won't have to do it by ourselves.
I'm not sure how mapStateToProps can confuse someone. We are talking about a state management library
I've seen some devs that misunderstood this because react
has a state
and redux
has a store
(at least that's how it was called in most of the tutorials and documentations).
this can be confusing to some people that are new to either react
or redux
.
Edit 2
It was a bit confusing due to the sentence 'it doesn't mean the wraped component is a Container.' Why is the wrapped component not a container? Isn't a component created by connect also a container?
I mean that the wrapped component that you wrote doesn't have to be a Container.
You can connect a "Presentation" component:
const Link = ({ active, children, onClick }) => {
if (active) {
return <span>{children}</span>
}
return (
<a
href=""
onClick={e => {
e.preventDefault()
onClick()
}}
>
{children}
</a>
)
}
// ...
export default connect(mapState, mapDispatch)(Link)
First of all these guidelines are not part of the bible
you should write code that is easy to reason about for YOU and your TEAM.
I think you are missing something, A redux Container is different than a react Container.
I mean, connect
will create the container for you, it doesn't mean the wraped component is a Container.
Basically you can export both versions from the same file, the Container (connected version) and the presentation version (the none connected one).
Another thing that usually throw people off, is the name of the function and argument of mapStateToProps
.
I prefer the name mapStoreToProps
as in
map the
redux
store to the component's props.
the name state
can be confusing when we are in the context of react
.
Edit
As a followup to your comment:
I totally didn't know these two are actually different. Could you please tell me about more details
They are different in the way that connect
is creating a "Container" for you.
connect
is a High Order Component that creates the Container Component for us with all the subscription logic + functions to pass portions of the store and action-creators to its children as props (mapStateToProps
& mapDispatchToProps
).
A "normal" Container is usually refers to a component that you write by hand, its often doesn't deal with how things should look but instead deal with certain logic of the app.
As for the other comments like
The connect HoC of react-redux just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified
As i mentioned above, this is partially true. It's not just injecting the properties into our component, its subscribing to the store, grabbing it from the Provider
(via context
) and its doing all these with optimizations in mind, so we won't have to do it by ourselves.
I'm not sure how mapStateToProps can confuse someone. We are talking about a state management library
I've seen some devs that misunderstood this because react
has a state
and redux
has a store
(at least that's how it was called in most of the tutorials and documentations).
this can be confusing to some people that are new to either react
or redux
.
Edit 2
It was a bit confusing due to the sentence 'it doesn't mean the wraped component is a Container.' Why is the wrapped component not a container? Isn't a component created by connect also a container?
I mean that the wrapped component that you wrote doesn't have to be a Container.
You can connect a "Presentation" component:
const Link = ({ active, children, onClick }) => {
if (active) {
return <span>{children}</span>
}
return (
<a
href=""
onClick={e => {
e.preventDefault()
onClick()
}}
>
{children}
</a>
)
}
// ...
export default connect(mapState, mapDispatch)(Link)
edited Dec 16 '18 at 14:04
answered Dec 16 '18 at 12:51


Sagiv b.gSagiv b.g
16.7k32258
16.7k32258
Thank you for your answer. But I don't understand this part : 'I think you are missing something, A redux Container is different than a react Container. I mean, connect will create the container for you, it doesn't mean the wraped component is a Container.' Yes, I totally didn't know these two are actually different. Could you please tell me about more details?
– Rhee
Dec 16 '18 at 13:04
I'm not sure howmapStateToProps
can confuse someone. We are talking about a state management library.
– Kyle Richardson
Dec 16 '18 at 13:05
1
Theconnect
HoC ofreact-redux
just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified.
– Kyle Richardson
Dec 16 '18 at 13:08
1
I've added some more details to answer some of the comments
– Sagiv b.g
Dec 16 '18 at 13:27
1
@Rhee I've answered you latest comment (Edit 2 section) Hope that's clarify it :)
– Sagiv b.g
Dec 16 '18 at 14:11
|
show 1 more comment
Thank you for your answer. But I don't understand this part : 'I think you are missing something, A redux Container is different than a react Container. I mean, connect will create the container for you, it doesn't mean the wraped component is a Container.' Yes, I totally didn't know these two are actually different. Could you please tell me about more details?
– Rhee
Dec 16 '18 at 13:04
I'm not sure howmapStateToProps
can confuse someone. We are talking about a state management library.
– Kyle Richardson
Dec 16 '18 at 13:05
1
Theconnect
HoC ofreact-redux
just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified.
– Kyle Richardson
Dec 16 '18 at 13:08
1
I've added some more details to answer some of the comments
– Sagiv b.g
Dec 16 '18 at 13:27
1
@Rhee I've answered you latest comment (Edit 2 section) Hope that's clarify it :)
– Sagiv b.g
Dec 16 '18 at 14:11
Thank you for your answer. But I don't understand this part : 'I think you are missing something, A redux Container is different than a react Container. I mean, connect will create the container for you, it doesn't mean the wraped component is a Container.' Yes, I totally didn't know these two are actually different. Could you please tell me about more details?
– Rhee
Dec 16 '18 at 13:04
Thank you for your answer. But I don't understand this part : 'I think you are missing something, A redux Container is different than a react Container. I mean, connect will create the container for you, it doesn't mean the wraped component is a Container.' Yes, I totally didn't know these two are actually different. Could you please tell me about more details?
– Rhee
Dec 16 '18 at 13:04
I'm not sure how
mapStateToProps
can confuse someone. We are talking about a state management library.– Kyle Richardson
Dec 16 '18 at 13:05
I'm not sure how
mapStateToProps
can confuse someone. We are talking about a state management library.– Kyle Richardson
Dec 16 '18 at 13:05
1
1
The
connect
HoC of react-redux
just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified.– Kyle Richardson
Dec 16 '18 at 13:08
The
connect
HoC of react-redux
just injects the properties you can request into your component. It returns a new component that is wrapped around your component so that it can update your component whenever the state you're interested in the redux store is modified.– Kyle Richardson
Dec 16 '18 at 13:08
1
1
I've added some more details to answer some of the comments
– Sagiv b.g
Dec 16 '18 at 13:27
I've added some more details to answer some of the comments
– Sagiv b.g
Dec 16 '18 at 13:27
1
1
@Rhee I've answered you latest comment (Edit 2 section) Hope that's clarify it :)
– Sagiv b.g
Dec 16 '18 at 14:11
@Rhee I've answered you latest comment (Edit 2 section) Hope that's clarify it :)
– Sagiv b.g
Dec 16 '18 at 14:11
|
show 1 more comment
mapStateToProps
will be called when store data changes. It will pass the returned object as new props for the component. This will not affect the component's state. If you'd like to set a new state after the component got its new props you need to use another lifecycle method: static getDerivedStateFromProps
(in earlier versions of react componentWillRecieveProps
). The object returned by static getDerivedStateFromProps
will be your new state.
https://reactjs.org/docs/state-and-lifecycle.html#adding-lifecycle-methods-to-a-class
connect()
will connect your component to the redux store. Withouth the connect function (of course) your mapStateToProps
will not work.
I'm not sure why react-redux named the API 'mapStateToProps'
We are talking about the store's state :)
add a comment |
mapStateToProps
will be called when store data changes. It will pass the returned object as new props for the component. This will not affect the component's state. If you'd like to set a new state after the component got its new props you need to use another lifecycle method: static getDerivedStateFromProps
(in earlier versions of react componentWillRecieveProps
). The object returned by static getDerivedStateFromProps
will be your new state.
https://reactjs.org/docs/state-and-lifecycle.html#adding-lifecycle-methods-to-a-class
connect()
will connect your component to the redux store. Withouth the connect function (of course) your mapStateToProps
will not work.
I'm not sure why react-redux named the API 'mapStateToProps'
We are talking about the store's state :)
add a comment |
mapStateToProps
will be called when store data changes. It will pass the returned object as new props for the component. This will not affect the component's state. If you'd like to set a new state after the component got its new props you need to use another lifecycle method: static getDerivedStateFromProps
(in earlier versions of react componentWillRecieveProps
). The object returned by static getDerivedStateFromProps
will be your new state.
https://reactjs.org/docs/state-and-lifecycle.html#adding-lifecycle-methods-to-a-class
connect()
will connect your component to the redux store. Withouth the connect function (of course) your mapStateToProps
will not work.
I'm not sure why react-redux named the API 'mapStateToProps'
We are talking about the store's state :)
mapStateToProps
will be called when store data changes. It will pass the returned object as new props for the component. This will not affect the component's state. If you'd like to set a new state after the component got its new props you need to use another lifecycle method: static getDerivedStateFromProps
(in earlier versions of react componentWillRecieveProps
). The object returned by static getDerivedStateFromProps
will be your new state.
https://reactjs.org/docs/state-and-lifecycle.html#adding-lifecycle-methods-to-a-class
connect()
will connect your component to the redux store. Withouth the connect function (of course) your mapStateToProps
will not work.
I'm not sure why react-redux named the API 'mapStateToProps'
We are talking about the store's state :)
edited Dec 16 '18 at 12:59
answered Dec 16 '18 at 12:53
messerbillmesserbill
3,1031225
3,1031225
add a comment |
add a comment |
The high level purpose is to seamlessly integrate Redux's state management into the React application. Redux revolves around the store where all the state exists. There is no way to directly modify the store except through reducers whom receive actions from action creators and for that to happen we need for an action to be dispatched from the action creator.
The connect()
function directly connects our components to the Redux store by taking the state in the Redux store and mapping it into a prop
.
This is power of Redux and its why we use it.
Lets say you are building a component called LaundryList
and you want it to render a laundry list. After you have wired up the Provider
in your "parent" component, I put it in quotes because technically Provider
is a component so it becomes the parent.
You can then import the connect()
function from react-redux
, pass it mapStateToProps
in order to get that laundry list from the Redux store into your LaundryList
component.
Now that you have your list of linens inside of the LaundryList
component you can start to focus on building a list of elements out of them like so:
class LaundryList extends Component {
render() {
console.log(this.props.linens);
return <div>LaundryList</div>;
}
}
That contains the list of linens object and for every list of linens inside of there we are going to return some jsx that is going to represent that linen on my list.
Back inside my laundry list component I will add a helper method inside the laundry list component called render list like so:
class LaundryList extends Component {
renderList() {
}
render() {
return <div>LaundryList</div>;
}
}
So this purpose of this helper method is to take the list of linens, map over them and return a big blob of jsx like so:
class LaundryList extends Component {
renderList() {
return this.props.linens.map((linen) => {
return (
);
});
}
render() {
return <div>LaundryList</div>;
}
}
add a comment |
The high level purpose is to seamlessly integrate Redux's state management into the React application. Redux revolves around the store where all the state exists. There is no way to directly modify the store except through reducers whom receive actions from action creators and for that to happen we need for an action to be dispatched from the action creator.
The connect()
function directly connects our components to the Redux store by taking the state in the Redux store and mapping it into a prop
.
This is power of Redux and its why we use it.
Lets say you are building a component called LaundryList
and you want it to render a laundry list. After you have wired up the Provider
in your "parent" component, I put it in quotes because technically Provider
is a component so it becomes the parent.
You can then import the connect()
function from react-redux
, pass it mapStateToProps
in order to get that laundry list from the Redux store into your LaundryList
component.
Now that you have your list of linens inside of the LaundryList
component you can start to focus on building a list of elements out of them like so:
class LaundryList extends Component {
render() {
console.log(this.props.linens);
return <div>LaundryList</div>;
}
}
That contains the list of linens object and for every list of linens inside of there we are going to return some jsx that is going to represent that linen on my list.
Back inside my laundry list component I will add a helper method inside the laundry list component called render list like so:
class LaundryList extends Component {
renderList() {
}
render() {
return <div>LaundryList</div>;
}
}
So this purpose of this helper method is to take the list of linens, map over them and return a big blob of jsx like so:
class LaundryList extends Component {
renderList() {
return this.props.linens.map((linen) => {
return (
);
});
}
render() {
return <div>LaundryList</div>;
}
}
add a comment |
The high level purpose is to seamlessly integrate Redux's state management into the React application. Redux revolves around the store where all the state exists. There is no way to directly modify the store except through reducers whom receive actions from action creators and for that to happen we need for an action to be dispatched from the action creator.
The connect()
function directly connects our components to the Redux store by taking the state in the Redux store and mapping it into a prop
.
This is power of Redux and its why we use it.
Lets say you are building a component called LaundryList
and you want it to render a laundry list. After you have wired up the Provider
in your "parent" component, I put it in quotes because technically Provider
is a component so it becomes the parent.
You can then import the connect()
function from react-redux
, pass it mapStateToProps
in order to get that laundry list from the Redux store into your LaundryList
component.
Now that you have your list of linens inside of the LaundryList
component you can start to focus on building a list of elements out of them like so:
class LaundryList extends Component {
render() {
console.log(this.props.linens);
return <div>LaundryList</div>;
}
}
That contains the list of linens object and for every list of linens inside of there we are going to return some jsx that is going to represent that linen on my list.
Back inside my laundry list component I will add a helper method inside the laundry list component called render list like so:
class LaundryList extends Component {
renderList() {
}
render() {
return <div>LaundryList</div>;
}
}
So this purpose of this helper method is to take the list of linens, map over them and return a big blob of jsx like so:
class LaundryList extends Component {
renderList() {
return this.props.linens.map((linen) => {
return (
);
});
}
render() {
return <div>LaundryList</div>;
}
}
The high level purpose is to seamlessly integrate Redux's state management into the React application. Redux revolves around the store where all the state exists. There is no way to directly modify the store except through reducers whom receive actions from action creators and for that to happen we need for an action to be dispatched from the action creator.
The connect()
function directly connects our components to the Redux store by taking the state in the Redux store and mapping it into a prop
.
This is power of Redux and its why we use it.
Lets say you are building a component called LaundryList
and you want it to render a laundry list. After you have wired up the Provider
in your "parent" component, I put it in quotes because technically Provider
is a component so it becomes the parent.
You can then import the connect()
function from react-redux
, pass it mapStateToProps
in order to get that laundry list from the Redux store into your LaundryList
component.
Now that you have your list of linens inside of the LaundryList
component you can start to focus on building a list of elements out of them like so:
class LaundryList extends Component {
render() {
console.log(this.props.linens);
return <div>LaundryList</div>;
}
}
That contains the list of linens object and for every list of linens inside of there we are going to return some jsx that is going to represent that linen on my list.
Back inside my laundry list component I will add a helper method inside the laundry list component called render list like so:
class LaundryList extends Component {
renderList() {
}
render() {
return <div>LaundryList</div>;
}
}
So this purpose of this helper method is to take the list of linens, map over them and return a big blob of jsx like so:
class LaundryList extends Component {
renderList() {
return this.props.linens.map((linen) => {
return (
);
});
}
render() {
return <div>LaundryList</div>;
}
}
answered Dec 23 '18 at 17:09


DanielDaniel
1,60521536
1,60521536
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53802181%2fi-cant-understand-why-components-with-connect-are-stateful-in-react%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bGRmO,kg4nLUI aEdL5OW5zJf,Y hi7aX3,zJCKPzIQ
"state" isn't a term specific to react, it's general to computer programming. It's a general term meaning remembered state of the system. en.wikipedia.org/wiki/State_(computer_science). Redux reducer state and component state are different things.
– Andy Ray
Dec 17 '18 at 2:26