How do I make a while loop with multiple conditions stop at a true condition?
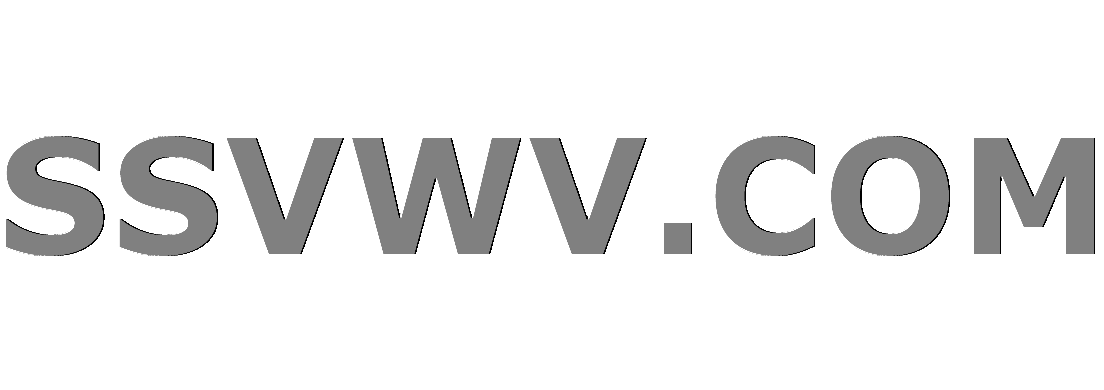
Multi tool use
I am supplying a while loop with the following
#!/bin/bash
number1=1
while [ -z "$number2" ] | [ "$number2" == 404 ] & [ "$number2" != 200 ] & [ "$number1" -lt 13 ]; do
#number2=$(some command which can actually get a number)
number2=200 # <<< e.g. a command that would return
let number1=number1+1
done
This is what I need to do
If
number2
is null do the loop
Ifnumber2
is 404 do the loop
Ifnumber2
is 200 don't do the loop
Do the loop untilnumber1
is 12
When I try the loop with number2=200
it doesn't stop. It seems I am having a challenge with having it to stop where number2
is 200
.
How do I write the statement such that it will stop the while loop when number2=200
or is there an alternative?
bash
add a comment |
I am supplying a while loop with the following
#!/bin/bash
number1=1
while [ -z "$number2" ] | [ "$number2" == 404 ] & [ "$number2" != 200 ] & [ "$number1" -lt 13 ]; do
#number2=$(some command which can actually get a number)
number2=200 # <<< e.g. a command that would return
let number1=number1+1
done
This is what I need to do
If
number2
is null do the loop
Ifnumber2
is 404 do the loop
Ifnumber2
is 200 don't do the loop
Do the loop untilnumber1
is 12
When I try the loop with number2=200
it doesn't stop. It seems I am having a challenge with having it to stop where number2
is 200
.
How do I write the statement such that it will stop the while loop when number2=200
or is there an alternative?
bash
What would happen if$number2
is 300 or 500?
– Kusalananda
Dec 14 '18 at 10:14
@Kusalananda$number2
is either null 404 or 200
– Bret Joseph
Dec 14 '18 at 10:39
add a comment |
I am supplying a while loop with the following
#!/bin/bash
number1=1
while [ -z "$number2" ] | [ "$number2" == 404 ] & [ "$number2" != 200 ] & [ "$number1" -lt 13 ]; do
#number2=$(some command which can actually get a number)
number2=200 # <<< e.g. a command that would return
let number1=number1+1
done
This is what I need to do
If
number2
is null do the loop
Ifnumber2
is 404 do the loop
Ifnumber2
is 200 don't do the loop
Do the loop untilnumber1
is 12
When I try the loop with number2=200
it doesn't stop. It seems I am having a challenge with having it to stop where number2
is 200
.
How do I write the statement such that it will stop the while loop when number2=200
or is there an alternative?
bash
I am supplying a while loop with the following
#!/bin/bash
number1=1
while [ -z "$number2" ] | [ "$number2" == 404 ] & [ "$number2" != 200 ] & [ "$number1" -lt 13 ]; do
#number2=$(some command which can actually get a number)
number2=200 # <<< e.g. a command that would return
let number1=number1+1
done
This is what I need to do
If
number2
is null do the loop
Ifnumber2
is 404 do the loop
Ifnumber2
is 200 don't do the loop
Do the loop untilnumber1
is 12
When I try the loop with number2=200
it doesn't stop. It seems I am having a challenge with having it to stop where number2
is 200
.
How do I write the statement such that it will stop the while loop when number2=200
or is there an alternative?
bash
bash
edited Dec 14 '18 at 13:35


SouravGhosh
457311
457311
asked Dec 14 '18 at 9:58


Bret JosephBret Joseph
759
759
What would happen if$number2
is 300 or 500?
– Kusalananda
Dec 14 '18 at 10:14
@Kusalananda$number2
is either null 404 or 200
– Bret Joseph
Dec 14 '18 at 10:39
add a comment |
What would happen if$number2
is 300 or 500?
– Kusalananda
Dec 14 '18 at 10:14
@Kusalananda$number2
is either null 404 or 200
– Bret Joseph
Dec 14 '18 at 10:39
What would happen if
$number2
is 300 or 500?– Kusalananda
Dec 14 '18 at 10:14
What would happen if
$number2
is 300 or 500?– Kusalananda
Dec 14 '18 at 10:14
@Kusalananda
$number2
is either null 404 or 200– Bret Joseph
Dec 14 '18 at 10:39
@Kusalananda
$number2
is either null 404 or 200– Bret Joseph
Dec 14 '18 at 10:39
add a comment |
2 Answers
2
active
oldest
votes
If number2 is null do the loop
If number2 is 404 do the loop
If number2 is 200 don't do the loop
Do the loop until number1 is 12
In other words, repeat as long as (number2 is null OR number2 = 404) AND (number2 != 200) AND (number1 <= 12)
. Note that you need some sort of grouping here, to make the precedence of AND and OR explicit. (In Bash, &&
and ||
operate from left to right, but often the AND-operator binds more strongly than an OR-operator.)
Though you didn't say what should happen for other values of number2
, so we might as well drop the first two conditions, since if number2
is null or 404, then it can't be 200. So we get (number2 != 200) AND (number1 <= 12)
.
Here,
while [ -z "$number2" ] | [ "$number2" == 404 ] & [ "$number2" != 200 ] & [ "$number1" -lt 13 ]; do ...
you have |
and &
instead of ||
and &&
. |
indicates a pipeline, and &
runs the preceding command in the background. So the above would run three commands in parallel: one pipeline with two tests, and another with one test, both in the background; and one test in the foreground. That doesn't make much sense. I mentioned &&
and ||
above, those are the logical condition operators in Bash.
The simplified form would be:
while [ "$number2" != 200 ] && [ "$number1" -le 12 ]; do ...
(You may also want to use somewhat more descriptive variable names than "number1" and "number2".)
Thanks.. let me go through the commands and see if i need more clarity
– Bret Joseph
Dec 14 '18 at 10:43
add a comment |
Logical operators in the shell are &&
and ||
. The &
and |
does very different things (starts a background task and sets up a pipe between two processes, respectively).
#!/bin/sh
number1=1
while [ "$number1" -le 12 ]; do
number2=$( some command )
case $number2 in
200)
break
;;
""|404)
# nothing
;;
*)
printf 'Unexpected: number2 = %sn' "$number2" >&2
exit 1
esac
number1=$(( number1 + 1 ))
done
The number in $number2
looks like a HTTP status code. Testing the value in a case
statement as above would allow you to select the correct action given any number of status codes without making the shell code an unwieldy mess of if
-statements. For example, the action for any client or server failure code could be triggered by the pattern 4??|5??
.
This also logically separates the semantics of the two variables. The number1
variable controls the number of iterations, while number2
is strictly for controlling the action to take based on the outcome of your mystery command.
ya the mystery command returns HTTP code and I want to do recheck if with another number if I get 404 again and stop if I get 200 and do something after
– Bret Joseph
Dec 14 '18 at 11:05
@BretJoseph In that case, you may want to do an additional check after the loop to make sure that you know why the loop terminated. It can terminate because of having run 12 times, or it may terminate because you got your 200 status.
– Kusalananda
Dec 14 '18 at 11:07
as long as it stops after the 200 status or re-loops if not its fine, I will make it do something before it breaks
– Bret Joseph
Dec 14 '18 at 11:10
add a comment |
Your Answer
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "106"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f487941%2fhow-do-i-make-a-while-loop-with-multiple-conditions-stop-at-a-true-condition%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
If number2 is null do the loop
If number2 is 404 do the loop
If number2 is 200 don't do the loop
Do the loop until number1 is 12
In other words, repeat as long as (number2 is null OR number2 = 404) AND (number2 != 200) AND (number1 <= 12)
. Note that you need some sort of grouping here, to make the precedence of AND and OR explicit. (In Bash, &&
and ||
operate from left to right, but often the AND-operator binds more strongly than an OR-operator.)
Though you didn't say what should happen for other values of number2
, so we might as well drop the first two conditions, since if number2
is null or 404, then it can't be 200. So we get (number2 != 200) AND (number1 <= 12)
.
Here,
while [ -z "$number2" ] | [ "$number2" == 404 ] & [ "$number2" != 200 ] & [ "$number1" -lt 13 ]; do ...
you have |
and &
instead of ||
and &&
. |
indicates a pipeline, and &
runs the preceding command in the background. So the above would run three commands in parallel: one pipeline with two tests, and another with one test, both in the background; and one test in the foreground. That doesn't make much sense. I mentioned &&
and ||
above, those are the logical condition operators in Bash.
The simplified form would be:
while [ "$number2" != 200 ] && [ "$number1" -le 12 ]; do ...
(You may also want to use somewhat more descriptive variable names than "number1" and "number2".)
Thanks.. let me go through the commands and see if i need more clarity
– Bret Joseph
Dec 14 '18 at 10:43
add a comment |
If number2 is null do the loop
If number2 is 404 do the loop
If number2 is 200 don't do the loop
Do the loop until number1 is 12
In other words, repeat as long as (number2 is null OR number2 = 404) AND (number2 != 200) AND (number1 <= 12)
. Note that you need some sort of grouping here, to make the precedence of AND and OR explicit. (In Bash, &&
and ||
operate from left to right, but often the AND-operator binds more strongly than an OR-operator.)
Though you didn't say what should happen for other values of number2
, so we might as well drop the first two conditions, since if number2
is null or 404, then it can't be 200. So we get (number2 != 200) AND (number1 <= 12)
.
Here,
while [ -z "$number2" ] | [ "$number2" == 404 ] & [ "$number2" != 200 ] & [ "$number1" -lt 13 ]; do ...
you have |
and &
instead of ||
and &&
. |
indicates a pipeline, and &
runs the preceding command in the background. So the above would run three commands in parallel: one pipeline with two tests, and another with one test, both in the background; and one test in the foreground. That doesn't make much sense. I mentioned &&
and ||
above, those are the logical condition operators in Bash.
The simplified form would be:
while [ "$number2" != 200 ] && [ "$number1" -le 12 ]; do ...
(You may also want to use somewhat more descriptive variable names than "number1" and "number2".)
Thanks.. let me go through the commands and see if i need more clarity
– Bret Joseph
Dec 14 '18 at 10:43
add a comment |
If number2 is null do the loop
If number2 is 404 do the loop
If number2 is 200 don't do the loop
Do the loop until number1 is 12
In other words, repeat as long as (number2 is null OR number2 = 404) AND (number2 != 200) AND (number1 <= 12)
. Note that you need some sort of grouping here, to make the precedence of AND and OR explicit. (In Bash, &&
and ||
operate from left to right, but often the AND-operator binds more strongly than an OR-operator.)
Though you didn't say what should happen for other values of number2
, so we might as well drop the first two conditions, since if number2
is null or 404, then it can't be 200. So we get (number2 != 200) AND (number1 <= 12)
.
Here,
while [ -z "$number2" ] | [ "$number2" == 404 ] & [ "$number2" != 200 ] & [ "$number1" -lt 13 ]; do ...
you have |
and &
instead of ||
and &&
. |
indicates a pipeline, and &
runs the preceding command in the background. So the above would run three commands in parallel: one pipeline with two tests, and another with one test, both in the background; and one test in the foreground. That doesn't make much sense. I mentioned &&
and ||
above, those are the logical condition operators in Bash.
The simplified form would be:
while [ "$number2" != 200 ] && [ "$number1" -le 12 ]; do ...
(You may also want to use somewhat more descriptive variable names than "number1" and "number2".)
If number2 is null do the loop
If number2 is 404 do the loop
If number2 is 200 don't do the loop
Do the loop until number1 is 12
In other words, repeat as long as (number2 is null OR number2 = 404) AND (number2 != 200) AND (number1 <= 12)
. Note that you need some sort of grouping here, to make the precedence of AND and OR explicit. (In Bash, &&
and ||
operate from left to right, but often the AND-operator binds more strongly than an OR-operator.)
Though you didn't say what should happen for other values of number2
, so we might as well drop the first two conditions, since if number2
is null or 404, then it can't be 200. So we get (number2 != 200) AND (number1 <= 12)
.
Here,
while [ -z "$number2" ] | [ "$number2" == 404 ] & [ "$number2" != 200 ] & [ "$number1" -lt 13 ]; do ...
you have |
and &
instead of ||
and &&
. |
indicates a pipeline, and &
runs the preceding command in the background. So the above would run three commands in parallel: one pipeline with two tests, and another with one test, both in the background; and one test in the foreground. That doesn't make much sense. I mentioned &&
and ||
above, those are the logical condition operators in Bash.
The simplified form would be:
while [ "$number2" != 200 ] && [ "$number1" -le 12 ]; do ...
(You may also want to use somewhat more descriptive variable names than "number1" and "number2".)
edited Dec 14 '18 at 11:37
answered Dec 14 '18 at 10:11


ilkkachuilkkachu
60.2k998171
60.2k998171
Thanks.. let me go through the commands and see if i need more clarity
– Bret Joseph
Dec 14 '18 at 10:43
add a comment |
Thanks.. let me go through the commands and see if i need more clarity
– Bret Joseph
Dec 14 '18 at 10:43
Thanks.. let me go through the commands and see if i need more clarity
– Bret Joseph
Dec 14 '18 at 10:43
Thanks.. let me go through the commands and see if i need more clarity
– Bret Joseph
Dec 14 '18 at 10:43
add a comment |
Logical operators in the shell are &&
and ||
. The &
and |
does very different things (starts a background task and sets up a pipe between two processes, respectively).
#!/bin/sh
number1=1
while [ "$number1" -le 12 ]; do
number2=$( some command )
case $number2 in
200)
break
;;
""|404)
# nothing
;;
*)
printf 'Unexpected: number2 = %sn' "$number2" >&2
exit 1
esac
number1=$(( number1 + 1 ))
done
The number in $number2
looks like a HTTP status code. Testing the value in a case
statement as above would allow you to select the correct action given any number of status codes without making the shell code an unwieldy mess of if
-statements. For example, the action for any client or server failure code could be triggered by the pattern 4??|5??
.
This also logically separates the semantics of the two variables. The number1
variable controls the number of iterations, while number2
is strictly for controlling the action to take based on the outcome of your mystery command.
ya the mystery command returns HTTP code and I want to do recheck if with another number if I get 404 again and stop if I get 200 and do something after
– Bret Joseph
Dec 14 '18 at 11:05
@BretJoseph In that case, you may want to do an additional check after the loop to make sure that you know why the loop terminated. It can terminate because of having run 12 times, or it may terminate because you got your 200 status.
– Kusalananda
Dec 14 '18 at 11:07
as long as it stops after the 200 status or re-loops if not its fine, I will make it do something before it breaks
– Bret Joseph
Dec 14 '18 at 11:10
add a comment |
Logical operators in the shell are &&
and ||
. The &
and |
does very different things (starts a background task and sets up a pipe between two processes, respectively).
#!/bin/sh
number1=1
while [ "$number1" -le 12 ]; do
number2=$( some command )
case $number2 in
200)
break
;;
""|404)
# nothing
;;
*)
printf 'Unexpected: number2 = %sn' "$number2" >&2
exit 1
esac
number1=$(( number1 + 1 ))
done
The number in $number2
looks like a HTTP status code. Testing the value in a case
statement as above would allow you to select the correct action given any number of status codes without making the shell code an unwieldy mess of if
-statements. For example, the action for any client or server failure code could be triggered by the pattern 4??|5??
.
This also logically separates the semantics of the two variables. The number1
variable controls the number of iterations, while number2
is strictly for controlling the action to take based on the outcome of your mystery command.
ya the mystery command returns HTTP code and I want to do recheck if with another number if I get 404 again and stop if I get 200 and do something after
– Bret Joseph
Dec 14 '18 at 11:05
@BretJoseph In that case, you may want to do an additional check after the loop to make sure that you know why the loop terminated. It can terminate because of having run 12 times, or it may terminate because you got your 200 status.
– Kusalananda
Dec 14 '18 at 11:07
as long as it stops after the 200 status or re-loops if not its fine, I will make it do something before it breaks
– Bret Joseph
Dec 14 '18 at 11:10
add a comment |
Logical operators in the shell are &&
and ||
. The &
and |
does very different things (starts a background task and sets up a pipe between two processes, respectively).
#!/bin/sh
number1=1
while [ "$number1" -le 12 ]; do
number2=$( some command )
case $number2 in
200)
break
;;
""|404)
# nothing
;;
*)
printf 'Unexpected: number2 = %sn' "$number2" >&2
exit 1
esac
number1=$(( number1 + 1 ))
done
The number in $number2
looks like a HTTP status code. Testing the value in a case
statement as above would allow you to select the correct action given any number of status codes without making the shell code an unwieldy mess of if
-statements. For example, the action for any client or server failure code could be triggered by the pattern 4??|5??
.
This also logically separates the semantics of the two variables. The number1
variable controls the number of iterations, while number2
is strictly for controlling the action to take based on the outcome of your mystery command.
Logical operators in the shell are &&
and ||
. The &
and |
does very different things (starts a background task and sets up a pipe between two processes, respectively).
#!/bin/sh
number1=1
while [ "$number1" -le 12 ]; do
number2=$( some command )
case $number2 in
200)
break
;;
""|404)
# nothing
;;
*)
printf 'Unexpected: number2 = %sn' "$number2" >&2
exit 1
esac
number1=$(( number1 + 1 ))
done
The number in $number2
looks like a HTTP status code. Testing the value in a case
statement as above would allow you to select the correct action given any number of status codes without making the shell code an unwieldy mess of if
-statements. For example, the action for any client or server failure code could be triggered by the pattern 4??|5??
.
This also logically separates the semantics of the two variables. The number1
variable controls the number of iterations, while number2
is strictly for controlling the action to take based on the outcome of your mystery command.
edited Dec 14 '18 at 11:03
answered Dec 14 '18 at 10:50


KusalanandaKusalananda
133k17254417
133k17254417
ya the mystery command returns HTTP code and I want to do recheck if with another number if I get 404 again and stop if I get 200 and do something after
– Bret Joseph
Dec 14 '18 at 11:05
@BretJoseph In that case, you may want to do an additional check after the loop to make sure that you know why the loop terminated. It can terminate because of having run 12 times, or it may terminate because you got your 200 status.
– Kusalananda
Dec 14 '18 at 11:07
as long as it stops after the 200 status or re-loops if not its fine, I will make it do something before it breaks
– Bret Joseph
Dec 14 '18 at 11:10
add a comment |
ya the mystery command returns HTTP code and I want to do recheck if with another number if I get 404 again and stop if I get 200 and do something after
– Bret Joseph
Dec 14 '18 at 11:05
@BretJoseph In that case, you may want to do an additional check after the loop to make sure that you know why the loop terminated. It can terminate because of having run 12 times, or it may terminate because you got your 200 status.
– Kusalananda
Dec 14 '18 at 11:07
as long as it stops after the 200 status or re-loops if not its fine, I will make it do something before it breaks
– Bret Joseph
Dec 14 '18 at 11:10
ya the mystery command returns HTTP code and I want to do recheck if with another number if I get 404 again and stop if I get 200 and do something after
– Bret Joseph
Dec 14 '18 at 11:05
ya the mystery command returns HTTP code and I want to do recheck if with another number if I get 404 again and stop if I get 200 and do something after
– Bret Joseph
Dec 14 '18 at 11:05
@BretJoseph In that case, you may want to do an additional check after the loop to make sure that you know why the loop terminated. It can terminate because of having run 12 times, or it may terminate because you got your 200 status.
– Kusalananda
Dec 14 '18 at 11:07
@BretJoseph In that case, you may want to do an additional check after the loop to make sure that you know why the loop terminated. It can terminate because of having run 12 times, or it may terminate because you got your 200 status.
– Kusalananda
Dec 14 '18 at 11:07
as long as it stops after the 200 status or re-loops if not its fine, I will make it do something before it breaks
– Bret Joseph
Dec 14 '18 at 11:10
as long as it stops after the 200 status or re-loops if not its fine, I will make it do something before it breaks
– Bret Joseph
Dec 14 '18 at 11:10
add a comment |
Thanks for contributing an answer to Unix & Linux Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2funix.stackexchange.com%2fquestions%2f487941%2fhow-do-i-make-a-while-loop-with-multiple-conditions-stop-at-a-true-condition%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PE5pu0 7YHhwbQ3AkWrFaLg tl,Pe1 H1hMKcP7jGI1P 7nAV pbO,Qe mh0YWCIQlJVZ1D1xhHk9xb
What would happen if
$number2
is 300 or 500?– Kusalananda
Dec 14 '18 at 10:14
@Kusalananda
$number2
is either null 404 or 200– Bret Joseph
Dec 14 '18 at 10:39